?
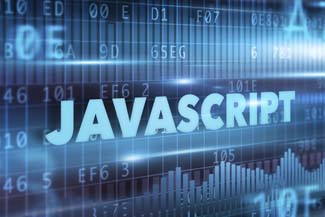
JavaScript is one of the most popular languages in the world. As software development moves towards cloud applications, JavaScript is the main option when the developer wants to run code on the client's browser or desktop. This is referred to as "client-side scripting." Most modern browsers include support for JavaScript functionality, so the developer doesn't need to force users to install third-party scripting software. Instead, the browser automatically understands the code and runs the client-side script without further installations from the developer. Conversely, if you wanted to run software on the browser that isn't supported by modern browsers, you would need to write and support a browser plugin, which can be much more difficult than just supporting JavaScript.
In this article, we'll learn about JavaScript, how to get started, and the way JavaScript and libraries have evolved to give programmers the ability to create asynchronous calls to web servers and create fast, creative user interfaces through the cloud and a user's local browser.
Why Use JavaScript?
If you code in backend software code such as PHP or C#, you are probably wondering why you would ever need JavaScript. Newer platforms integrate well with JavaScript libraries, but you can use backend, server-side code for much of the interface presentation and processing in the browser. There are several reasons to use JavaScript.
First, you can create asynchronous calls to a web server without forcing the entire page to reload. In older coding days, when you sent just one input back to the server, the entire page content would post back to the server. The user would have to wait for the page to post to the server, the server would process the response, and then the server would send another page back to the browser. This could take a few seconds, and the result could mean that the user needed several minutes to get through a checkout section of your ecommerce site or create an account on the server. With JavaScript and Ajax, you can submit a small part of your web form and post the response back to the user without making the entire page load. This means that the user can perform other actions on your site without waiting for the process to respond from the server.
Next, JavaScript is much faster than server-side code for basic UI functionality. JavaScript is better for drop-down menus or popups when the user hovers over an item. These little effects are much too time-consuming and tedious for server-side code. When a user clicks a menu item and a sub-menu displays, you can use JavaScript for a fast drop-down response. To use server-side script, you'd have to send the click event back to the server, process the results, and then reload the entire menu back to the user.
Finally, you can also use JavaScript to validate input from a form. For instance, suppose you want to validate that the user has entered a first name in your form. You don't want to waste server processing time by sending back blank input and then returning an error to the user. This would be time-consuming and a waste of time for your users. You can use JavaScript to verify that the user has entered a first name before sending input back to the server. This process is much quicker for the user, and it leaves a better user experience for the user instead of making them wait to find out if they filled out the form properly.
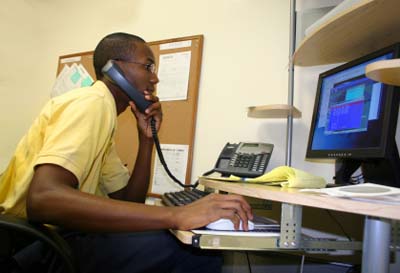
JavaScript is used for several current user interface (UI) products. If you install any third-party UI elements on your website, the third-party elements are likely using JavaScript. For instance, a slider that toggles images from one slide to the next uses JavaScript to change the images every few seconds. The UI element designer sets the JavaScript timer, and makes a call to the JavaScript function that changes the images. This is just one example of the way JavaScript is used in UI elements (including the drop-down menu example previously). When you hover over an image and a larger image is displayed, this is another example of JavaScript.
What Supports JavaScript?
JavaScript is supported by most major browsers. One issue with using JavaScript over other server-side languages is that JavaScript depends on the user's browser and the operating system. What works on a Windows Internet Explorer computer might not work on a Linux computer running Firefox. This can be an issue when you're creating public platforms that are meant to reach as many people as possible. For instance, if you run an ecommerce site, you don't want to limit the amount of people who can run your site simply because your JavaScript code doesn't run on a group of user's computers. This can be one of the downfalls of JavaScript.
When using basic JavaScript, you can be sure that most modern browsers support JavaScript code. The only issue is that some JavaScript commands are slightly different in Internet Explorer than they are in Firefox and Chrome. You'll notice that most large websites have a JS library for IE and a library for other browsers. This is due to these slight coding differences.
JavaScript is supported by the big browsers including Chrome, Firefox, Safari, and Internet Explorer. You can use tools such as Google Analytics to determine the popular browsers used on your site. Identify the common browsers used on your site and then ensure that your JavaScript libraries are compatible with common browsers. You want to limit your incompatibility issues as much as possible. For instance, Internet Explorer version 6 was included with Windows XP and supported years ago. There are still many people who still use this version of IE, but the browser is incompatible with most JS libraries used today.
When Not to Use JavaScript
Even though JavaScript is a part of any coder's arsenal, there are some times you don't want to use JavaScript. First, JavaScript is still not perfected for mobile devices. This means that you don't want to make a mobile site heavy with JavaScript programming. JavaScript libraries can use too many resources on older mobile devices, and some older mobile devices don't understand newer JavaScript coding.
Next, JavaScript is not always secure. There are several validation and coding mistakes that make your website vulnerable to SQL injection or cross-site hacking due to JavaScript scripts. When you run code on a user's computer, it means you have access to the local machine. This can then mean your visitors are vulnerable if the JavaScript is not coded properly. For instance, if you use JavaScript input to then write the input to the user's browser, the hacker can use this vulnerability to create XSS scripts that write content into server input. The input can then be passed to your server in the form elements, which in turn can execute SQL on the database server. XSS and SQL injection can get very complex, but these two hacking sources can stem from poor JavaScript coding design.
Another issue with JavaScript is that it isn't well understood by bots. When you build websites meant for the public, you probably want code that's easily crawled and understood by search engine bots such as Google, Bing or Yahoo. Google has been making efforts to crawl and understand JavaScript code and how it relates to a user interface and user experience elements. But this is newer technology and several elements in JavaScript are still in beta with search engines. In other words, if you want to make a site that is easily understood by search engines, you should limit JavaScript to only when you need it. Chances are that you'll need JavaScript at some point in your website coding, but you don't always need it when creating HTML elements.
You can potentially use JavaScript in all of your elements, but you should always limit its use to when you need it or when it makes a better user interface. You should highly consider JavaScript before you implement it. Is it better for users? Will it make the site faster and improve the user experience? Will it be more beneficial than server-side scripting? All of these questions should be answered before you decide to implement any type of code on your site. Also, consider the security benefits and losses when using JavaScript, and make sure you consider any cross-script hacking before you implement interaction between JavaScript and your SQL commands.
Variable Naming Rules and JavaScript Data Types
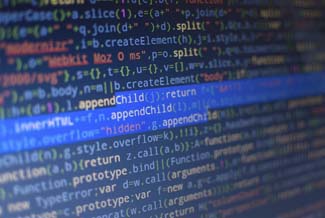
The start of any language is learning variables and how to name those variables. Most developers have a naming scheme they use to identify variable data types. When another coder reads your code, the backup coder should be able to quickly identify data types using variable naming rules. These naming rules help you work with data without causing errors on your website pages. In this lesson, we'll start off showing you how to create JS variables, work with data, and perform some basic JavaScript functionality.
Creating Basic JavaScript Variables
JavaScript variables are specified using the "var" keyword. If you have experience with other languages, you're probably used to defining the data type that will be stored in a variable before you actually store the variable. JavaScript just uses an open container instead of forcing you to define a data type.
The following code is an example of defining a variable in JavaScript.
var x = 10;
var y = 12;
The above code defines two variables. Notice that the code doesn't define that both variables are integers. The definition or data types are defined by assigning integers to the variable names. In the above code, the first variable name is "x" and the value assigned to the variable is "10." The second variable name is "y" and the assigned integer is "12." The semicolon after each JavaScript expression defines the end of the statement.
You can also do the same with string values. The following code defines a string variable.
var name = "Jennifer";
In the same way an integer was defined by assigning a number to a variable, the above code defines a string variable. The variables name is "name" and the value assigned to the variable is "Jennifer." You'll use a number of variables in your JavaScript scripts.
You don't always need to know what value to assign a variable. You can also define a variable with no assignment. The following code defines the variable "name" without giving it a string expression assignment.
var name;
In the above expression, you could technically assign the variable "name" to an integer value, but this goes against naming standards. Usually, when you name a variable, you give it a name that lets another coder know what data type is contained later in the code. These type of naming standards make it easier for a coder to understand as they browse your code for the first time.
With the variable name defined, you can later assign it a value. The following code assigns "name" with a string value.
name = "Jennifer";
Notice that the "var" keyword isn't used in this instance, because "var" defines the variable name and it's already been defined. The difference between defining a variable and assigning it a variable is important when you're coding in any language. A mistake can mean a syntax error in your code, which stops execution of JavaScript on the page and breaks your web pages.
Using Variables to Calculate Values
In the previous section, we discussed how to make variables, but you usually need to manipulate or evaluate these variables within your JS statements.
Let's take the previous two integer values we defined earlier.
var x = 10;
var y = 12;
You have two variables, but they don't do anything. We can calculate the sum of both of these variables by using the plus sign to add the two together. Once you have the sum of the two values, you need to assign this new value to a new variable. The following code shows you how to do this.
var x = 10;
var y = 12;
var z = x + y;
Notice the last variable adds both x and y, so the result is contained in the variable "z." The value of z is now 22. You can even use the z variable to then perform a calculation again and assign the new value to yet another variable. Take a look at the following code.
var x = 10;
var y = 12;
var z = x + y;
var w = z + x;
You can see how long JS scripts can get convoluted and hard to read. In most cases, developers run the code to verify the values are calculating properly without any logic errors. The above code assigns the sum of x and y to z, which calculates to 22. The value 22 is then added to x, which is still a value of 10. The sum totals to 32 and this value is assigned to the "w" variable.
You can also use the plus operator to combine strings. For instance, the following code combines strings together.
var firstName = "Joe";
var lastName = " Smith";
var fullName = firstName + lastName;
The result is "Joe Smith" assigned to the variable fullName.
There are a few rules when naming variables in JavaScript. You can't just give them any name. The following are the rules when you decide on a name for a variable:
-
The variable name must begin with a letter
-
The variable name can start with "$" or "$_" but these usually indicate an Ajax variable name, so most standards don't use these characters for naming variables unless they are Ajax.
-
Variable names can also contain numbers or underscores.
-
Variable names are case sensitive, which means that "Variable," "variable," and "VarIAble" are all different variables in a script.
-
You can't use reserved JavaScript keywords as variable names.
JavaScript Operators
In the previous section, you saw the plus sign used to add numbers or concatenate strings. There are several other operators you can use to perform calculation on values or variables.
The asterisk multiplies values. The following code shows you how to multiply.
var x = 3;
var y = 4;
var z = x * y;
The result is that z is assigned the value 12.
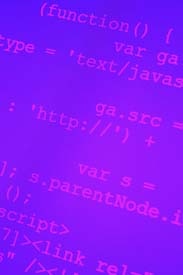
You'll also use division operators from time to time. The following performs the same action as the above code, but instead it performs division.
var x = 12;
var y = 4;
var z = x / y;
The above result is that z is assigned the value 3.
You can also use the above statements to practice with the subtraction operator or (-) the modulus operator or (%), which divides two numbers and returns the remainder. For instance, the following code uses the modulus operator.
var x = 12;
var y = 5;
var z = x % y;
12/5 does not return an even number, so the modulus operator returns "2" in this example.
You can also increment and decrement your numbers by one automatically. Instead of writing out the calculation to subtract or add 1 to a number, you can use the increment operator (++) or the decrement operator (--). The following code shows you an example.
var x = 12;
var z = x++;
The above code increments 1 to the x operator and assigns the value to the variable z. In this example, z is assigned the value 13.
JavaScript also has an "undefined" state where variables are returned as undefined when there is an error or the variable was never assigned a value. For instance, we learned that we can define a variable without assigning a value. The following code is from a previous section.
var name;
If we decided to print the above variable to the screen, the value "undefined" would be returned. Be careful with undefined variables. If you attempt to calculate a function or expression, the entire expression will result in an "undefined" returned value. For instance, the following code returns "undefined."
var name;
var result = name + 9;
Because name is never given a value, it's still undefined. When you try to make a calculation, the result is undefined. Be careful when you work with JS variables where you define names but decide to assign values later in the code. Most developers assign a 0 or some value to an integer or string when it's defined but you don't know the value of the variable just yet.